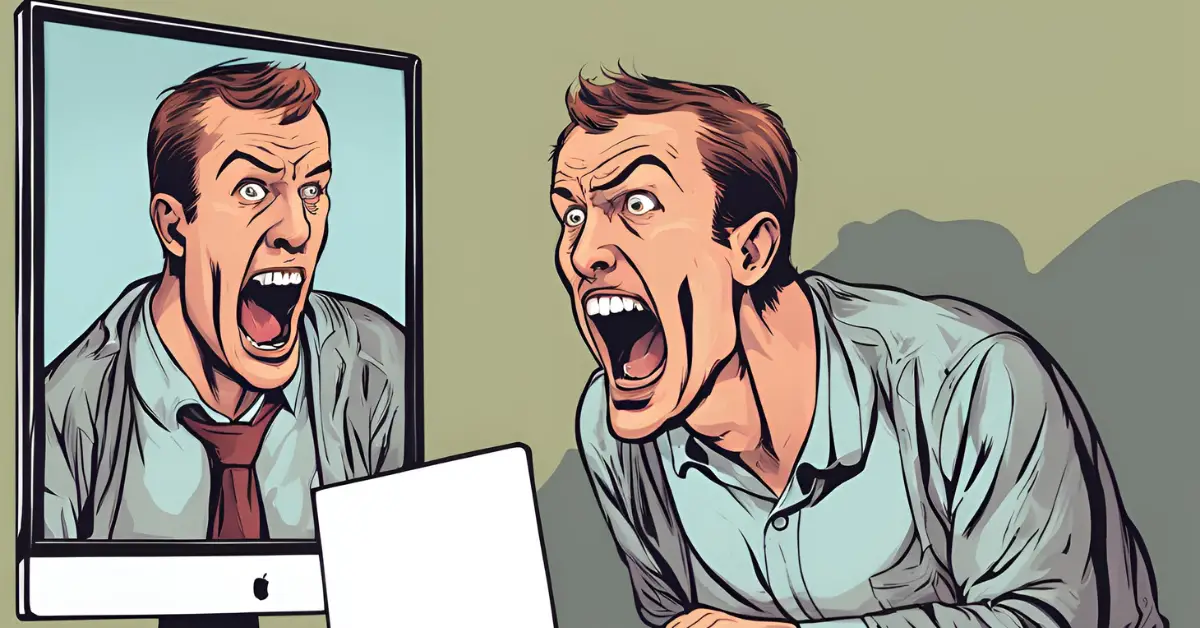
Variables are the building blocks of programming and are essential for managing data within any software application. Whether you’re just starting your coding journey. Or you’re a seasoned software testing professional looking to deepen your understanding of Data Structures and Algorithms (DSA). Grasping the concept of variables is fundamental.
In this article, we’ll explore what variables are. We will also discuss their types and their significance in DSA. You will see practical examples and best practices. By the end, you’ll have a solid foundation for utilizing variables effectively in your programming endeavors.
What Are Variables?
At its core, a variable is a symbolic name associated with a value. Think of it as a container that holds data that can change during the execution of a program. Variables allow programmers to store, modify, and retrieve data efficiently.
Why Are Variables Important?
Variables play a crucial role in programming for several reasons:
- Data Storage: They provide a way to store information that can be used and modified throughout a program.
- Flexibility: They allow programs to handle varying data inputs without requiring a complete rewrite of the code.
- Readability: Well-named variables enhance code readability, making it easier for developers to understand the purpose of the data being manipulated.
Analogy: Variables as Containers
Imagine variables as labeled containers in a warehouse. Each container (variable) can hold a different item (value), and you can easily retrieve or change the contents as needed. This analogy helps simplify the concept of how variables work in programming.
Types of Variables
In programming, variables can be categorized into different types based on several criteria, such as scope, lifetime, and data type. Let’s break down these categories.
1. Based on Scope
Type | Description |
---|---|
Local | Variables declared within a function or block. They can only be accessed within that function. |
Global | Variables declared outside any function. They can be accessed anywhere in the program. |
Static | Variables that retain their value even after the function in which they are declared exits. |
Instance | Variables associated with a specific instance of a class in Object-Oriented Programming (OOP). |
2. Based on Lifetime
Type | Description |
---|---|
Temporary | Variables created temporarily during a function call. They cease to exist once the function exits. |
Permanent | Variables that persist throughout the program’s execution. |
3. Based on Data Type
Data Type | Description |
---|---|
Integer | Represents whole numbers (e.g., int x = 10; ). |
Float | Represents decimal numbers (e.g., float y = 5.5; ). |
Character | Represents single characters (e.g., char c = 'A'; ). |
String | Represents sequences of characters (e.g., String name = "John"; ). |
Boolean | Represents true/false values (e.g., boolean isActive = true; ). |
Using Variables in DSA
In the context of Data Structures and Algorithms, variables serve various roles. Here’s how they fit into the bigger picture:
1. Data Structures
Variables are integral to creating and managing data structures. For example, when implementing a linked list, each node typically contains variables. These variables store the data and a pointer to the next node.
Example: Linked List Node
class Node {
int data; // Variable to hold data
Node next; // Variable to point to the next node
}
2. Algorithm Implementation
Variables are crucial in algorithms to store intermediate results, counters, and state information. For instance, in a sorting algorithm like QuickSort, variables are used to track the pivot element and indices for partitioning.
Example: QuickSort
void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high); // Variable to store pivot index
quickSort(arr, low, pivotIndex - 1); // Recursive call
quickSort(arr, pivotIndex + 1, high); // Recursive call
}
}
Best Practices for Using Variables
To ensure your code is efficient and maintainable, follow these best practices when working with variables:
1. Use Descriptive Names
Choose meaningful names that convey the purpose of the variable. For example, instead of int x;
, use int totalScore;
.
2. Keep Scope Minimal
Limit the scope of your variables to where they are needed. This reduces complexity and potential errors in your code.
3. Initialize Variables
Always initialize your variables before using them. Uninitialized variables can lead to unpredictable behavior.
4. Consistent Data Types
Be consistent with data types throughout your code to avoid type-related errors and improve readability.
5. Avoid Magic Numbers
Instead of using hard-coded numbers directly in your code, assign them to variables with meaningful names. For example:
// Instead of this:
if (age >= 18) { ... }
// Use this:
final int LEGAL_AGE = 18;
if (age >= LEGAL_AGE) { ... }
Conclusion
Variables are essential components in programming, particularly in Data Structures and Algorithms. They allow developers to store and manipulate data efficiently, making them foundational to writing effective code. By understanding the types of variables, software testing professionals can enhance their coding skills. Following best practices for variable use contributes to more robust software development.
As you continue your journey into DSA, remember the importance of variables in structuring your code and managing data effectively. With this knowledge, you’ll be better equipped to tackle complex problems and improve your programming proficiency.
FAQs
1. What are variables in programming? Variables are symbolic names associated with values that allow programmers to store and manipulate data in their code.
2. What is the difference between local and global variables? Local variables are accessible only within the function where they are declared. Global variables can be accessed from anywhere in the program.
3. Why is it important to initialize variables? Initializing variables prevents undefined behavior and ensures your program runs as expected by providing a known starting value.
4. How do I choose meaningful variable names? Choose names that describe the purpose or content of the variable. For example, totalAmount
is more descriptive than x
.
5. What are some common data types for variables? Common data types include integers, floats, characters, strings, and booleans, each serving different purposes in a program.
With this detailed guide on variables, you’re now better equipped to understand their role in programming and DSA. Embrace the power of variables to elevate your coding skills and improve your software testing capabilities!
Subscribe to QABash Weekly 💥
Dominate – Stay Ahead of 99% Testers!