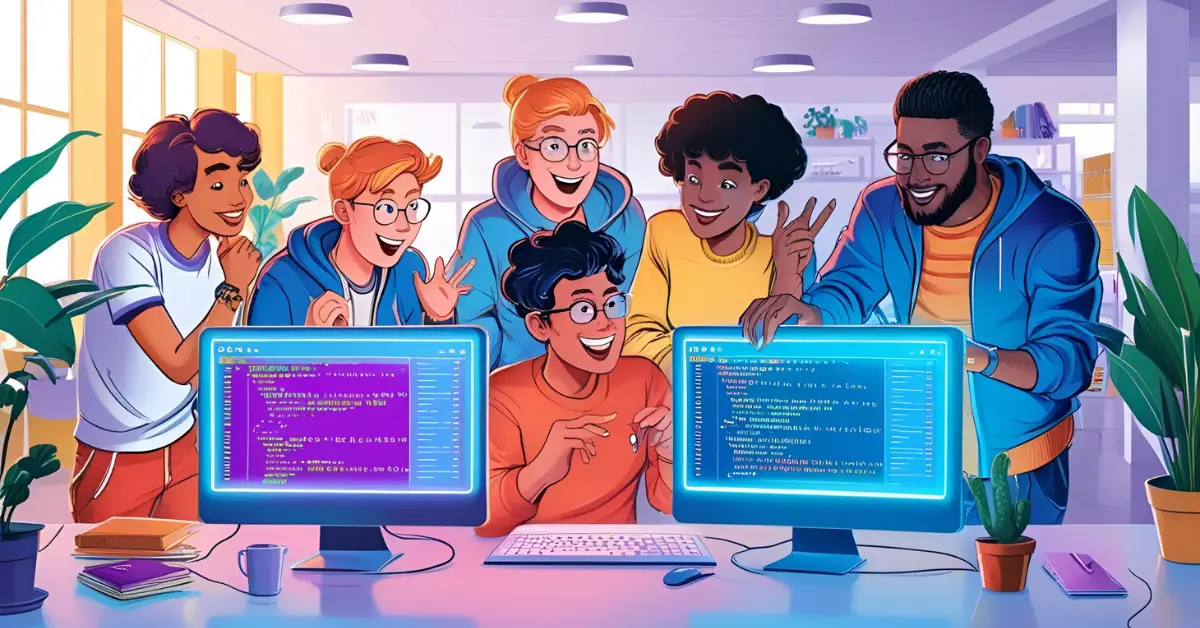
Why the Two Sum Problem Matters?
If youโre just starting your coding journey, youโve probably heard of the โTwo Sumโ problem. Itโs not just a common interview question โ itโs a perfect gateway into the world of algorithms and problem-solving.
The Two Sum problem asks a simple question:
Given an array of integers, can you find two numbers that add up to a specific target?
Sounds easy, right? But as you dig in, youโll uncover powerful lessons about data structures, efficiency, and coding best practices. In fact, this problem is asked in interviews by top tech companies like Google, Amazon, and Microsoft.
By the end of this guide, youโll not only understand how to solve it โ youโll know how to solve it like a pro.
๐งฉ What is the Two Sum Problem?
๐ Problem Statement
Given an array of integers
nums
and an integertarget
, return the indices of the two numbers such that they add up to the target.
๐งพ Example:
Input: nums = [2, 7, 11, 15], target = 9
Output: [0, 1]
Explanation: nums[0] + nums[1] = 2 + 7 = 9
๐ฅ Constraints:
- Each input has exactly one solution.
- You may not use the same element twice.
- You can return the answer in any order.
๐ ๏ธ Approaches to Solve the Two Sum Problem
Letโs explore different ways to solve it โ from brute force to optimal.
๐ Approach 1: Brute Force (For Absolute Beginners)
This is the most straightforward method. Try every possible pair of numbers and check if they sum up to the target.
Example usage
โ Pros:
- Easy to understand
- Good for learning nested loops
โ Cons:
- Time complexity: O(nยฒ)
- Not scalable
โก Approach 2: Using a Hash Map (Optimal & Efficient)
This is the most efficient and industry-preferred solution.
โ Idea:
Store each numberโs complement (target โ num) in a hash map. As you iterate, check if the complement exists.
๐งโ๐ป Code:
โฑ Time and Space Complexity:
- Time: O(n)
- Space: O(n)
๐ง Why This Works:
Hash maps (dictionaries) allow O(1) average time complexity for lookups โ making the solution lightning fast even for large inputs.
๐ก Practical Examples to Solidify Your Understanding
Example 1:
nums = [3, 2, 4], target = 6
Output: [1, 2] # 2 + 4 = 6
Example 2:
nums = [3, 3], target = 6
Output: [0, 1] # 3 + 3 = 6
๐ Try It Yourself:
Want to practice live? Use platforms like:
๐ง Expert Tips and Best Practices
- Always consider edge cases like duplicates and negative numbers.
- Practice on paper first โ it builds logic without relying on a compiler.
- Explain your thought process out loud โ crucial for coding interviews.
- Use meaningful variable names (e.g.,
complement
,index_map
) to improve code readability.
โ ๏ธ Common Mistakes to Avoid
- Using the same index twice
Always ensure youโre not pairing the same element with itself. - Assuming the array is sorted
Many beginners mistakenly try binary search. Unless sorted, this wonโt work. - Ignoring dictionary updates
If youโre not updating the hash map correctly, it may miss valid pairs. - Overthinking the problem
Itโs a simple pattern-based question. Donโt complicate it.
Frequently Asked Questions (FAQs)
Q1: Can I solve it without using extra space?
Yes, by sorting and using the two-pointer technique, but youโll need to track original indices, which adds complexity.
Q2: What if there are multiple solutions?
The standard problem assumes exactly one solution. But if modified, you can return all pairs that match.
Q3: Is this problem asked in interviews?
Absolutely! Itโs often the first question asked to test your fundamentals and thought process.
Whatโs Next?
You just tackled one of the most iconic algorithm problems in computer science! The Two Sum problem is more than a coding puzzle โ itโs a lesson in efficiency, problem-solving, and thinking like a developer.
Your Next Steps:
- Try solving Three Sum and Four Sum problems.
- Practice hash map and array manipulation techniques.
- Participate in daily coding challenges on LeetCode or Codeforces.
- Learn time-space tradeoffs to optimize further.
Subscribe to QABash Weekly ๐ฅ
Dominate โ Stay Ahead of 99% Testers!