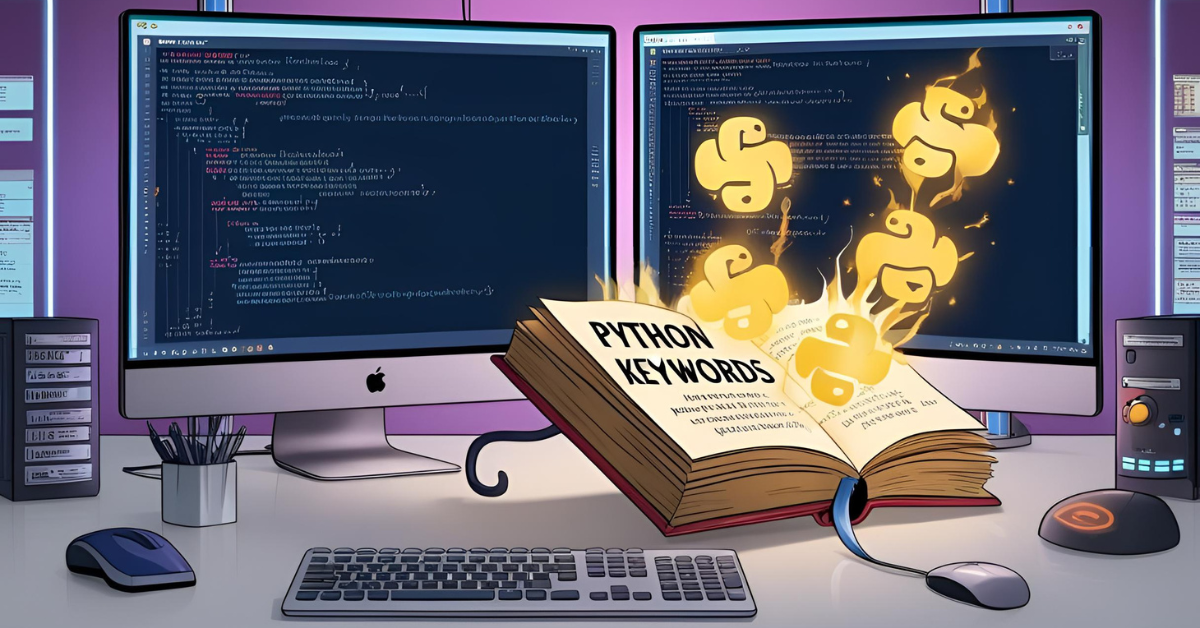
Wait… What Are These ‘Magic Words’ in Python?
Let’s imagine you’re learning a new language — say French. You can’t just randomly make up words and expect others to understand you, right? Well, Python’s the same. It has its own special set of reserved words, called keywords, and if you misuse them, Python gets confused.
In Python, keywords are the building blocks of every script. They’re like grammar rules — but cooler — because they control how your program runs, makes decisions, and handles errors.
If you’re a beginner Pythonista or even a curious SDET dipping your toes into scripting, mastering keywords is non-negotiable.
Let’s break them down — ELI5 style. 🤓
🧠 What Are Python Keywords, Exactly?
In the simplest terms:
Python keywords are reserved words that have a predefined meaning in the language and cannot be used as names for variables, functions, or anything else.
They’re kind of like the VIPs of Python — you can’t mess with them. Each keyword plays a role in shaping how your code behaves.
And yes, Python is picky — these keywords are case-sensitive, so if
works, but If
doesn’t.
📚 Why Are Keywords So Important?
Without keywords, your Python script would be like a sentence without grammar. Here’s what they do:
🔁 Control Flow
Keywords like if
, for
, and while
help Python decide what to do next — conditions, loops, etc.
🧱 Structure
They define the architecture of your code — def
for functions, class
for classes, and so on.
🛠️ Built-In Operations
Some keywords like return
, yield
, or raise
help you control logic, data, and errors precisely.
🗂️ All Python Keywords (as of Python 3.14)
Here’s a clean table with all 35 Python keywords and what they do — think of it as your cheat sheet:
Keyword | Description |
---|---|
False | Boolean value for false |
None | Represents null or no value |
True | Boolean value for true |
and | Logical AND |
as | Alias creation during imports |
assert | Debugging aid |
async | Declares an asynchronous function |
await | Waits for an asynchronous call |
break | Exits a loop early |
class | Declares a class |
continue | Skips to the next loop iteration |
def | Defines a function |
del | Deletes an object |
elif | Else if — part of a conditional |
else | Final option in a conditional |
except | Handles exceptions |
finally | Runs no matter what, post-exception |
for | Starts a loop |
from | Imports parts of a module |
global | Declares global variables |
if | Starts a conditional |
import | Imports modules or libraries |
in | Checks membership in a list, string, etc. |
is | Checks object identity |
lambda | Anonymous function |
nonlocal | Refers to outer-scope variable (but not global) |
not | Logical NOT |
or | Logical OR |
pass | Placeholder — does nothing |
raise | Raises an error |
return | Returns a value from a function |
try | Starts exception handling block |
while | Starts a while loop |
with | Simplifies file or resource handling |
yield | Returns generator output |
🧪 Explain Like I’m 5 (ELI5)
Let’s say you’re baking cookies (yum 🍪).
if
= “If the dough is sticky, add more flour.”for
= “For every cookie, put one chocolate chip on top.”def
= “Define how to bake cookies.”return
= “Return a tray full of baked cookies.”
See? Keywords = instructions. Your program follows them just like a recipe.
✅ Pro Tips for Using Keywords Like a Python Pro 🧠
📝 Tip 1: Learn by Categorizing
Group keywords by their function — loops, conditionals, functions, etc. It’ll help you memorize faster.
🧪 Tip 2: Use keyword
Module in Python
Type this:import keyword
print(keyword.kwlist)
This prints the latest list of keywords your version of Python supports. Super handy!
🚀 Tip 3: Practice in Real Code
Build tiny scripts: calculator, todo app, login system — and challenge yourself to use every keyword at least once.
🧹 Tip 4: Don’t Fight the Syntax
Trying to name a variable class
or if
will throw errors. Python won’t allow it — it’s like naming your dog “Password123”.
📅 Tip 5: Stay Updated
Python updates may add or deprecate keywords. Always check the official Python docs when switching versions.
⚠️ Common Mistakes Beginners Make
🚫 Using keywords as variable names
def = 10 # ❌ Nope.
🚫 Capitalizing keywords
If True: print("Hello") # ❌ Wrong.
🚫 Confusing is
with ==
Use is
to compare identities, ==
to compare values.
🚫 Not understanding pass
It’s not “do nothing forever.” It’s “skip this for now” — like a placeholder.
💡 Expert Advice: Master yield
and async
Two of Python’s most powerful (and confusing) keywords are yield
and async
. Once you’re comfortable with basics:
yield
helps you build memory-efficient generators (hello, data streams!).async
lets you write non-blocking, super-fast code — essential for APIs and automation tools.
Both are 🔥 for building scalable systems.
Wrapping Up: Know the Rules to Break Them
Learning Python keywords isn’t about memorization — it’s about fluency. Once you know what each one does and how it fits into the flow of a program, writing Python becomes natural.
Subscribe to QABash Weekly 💥
Dominate – Stay Ahead of 99% Testers!