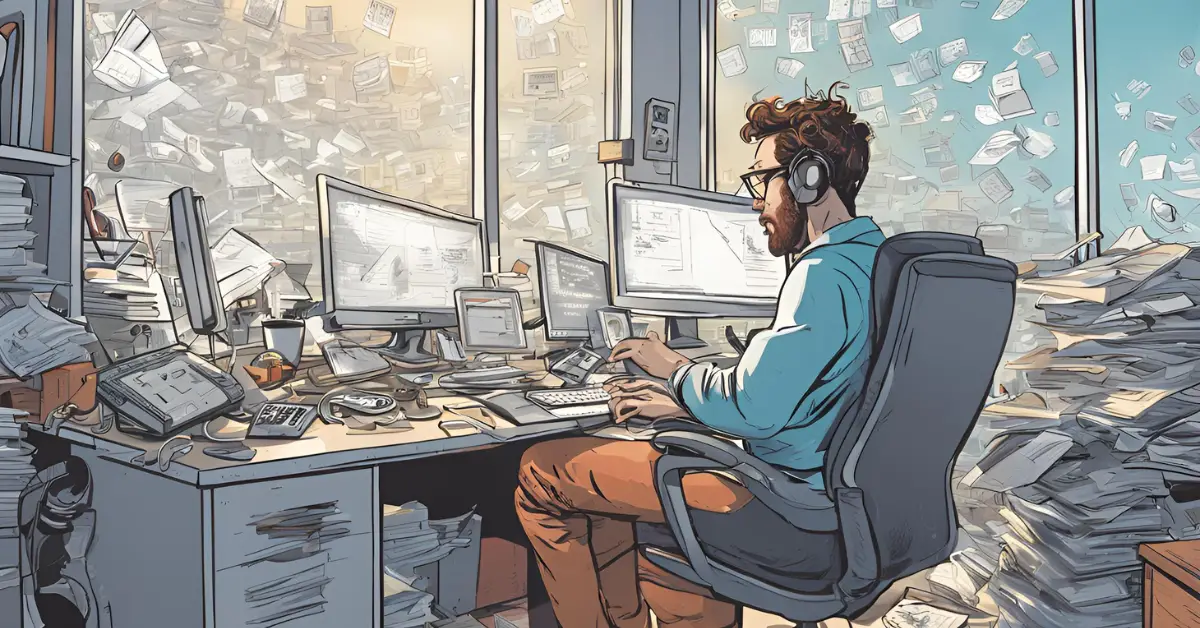
Data types are fundamental concepts in programming that define the kind of data a variable can hold. They play a crucial role in determining how the data is stored, manipulated, and interpreted. Understanding data types is essential for software testing professionals, as it influences everything from code performance to error detection.
Let’s explore the various data types, their classifications, and their significance in programming and data structures. I’ll also provide examples and tables to help clarify complex concepts. By the end, you’ll have a solid grasp of data types and how they impact your programming journey.
What Are Data Types?
In programming, a data type specifies the type of data that a variable can store. This information is crucial because it dictates how the programming language will interpret the data. For instance, a variable defined as an integer will only accept whole numbers. A variable defined as a string will handle sequences of characters.
Why Are Data Types Important?
- Memory Management: Data types help allocate appropriate amounts of memory, optimizing performance.
- Type Safety: They prevent errors by enforcing rules about how data can be used.
- Data Manipulation: Knowing the data type allows programmers to use appropriate techniques for data manipulation.
- Code Readability: Clearly defined data types enhance code readability and maintainability.
Analogy: Data Types as Containers
Think of data types as containers with specific shapes. A square container can only hold square objects. Similarly, a variable defined as an integer can only hold integer values. This analogy helps simplify the concept of how data types operate in programming.
Classifying Data Types
Data types can be broadly classified into two categories: Primitive Data Types and Composite Data Types.
1. Primitive Data Types
Primitive data types are the most basic forms of data. They are not composed of other data types and are predefined by programming languages. Here’s a breakdown of common primitive data types:
Data Type | Description | Example |
---|---|---|
Integer | Whole numbers, both positive and negative | int x = 10; |
Float | Decimal numbers, allowing fractions | float y = 5.5; |
Character | Single letters or symbols | char c = 'A'; |
Boolean | Represents true or false values | boolean isActive = true; |
Double | Double-precision floating-point numbers | double pi = 3.14; |
2. Composite Data Types
Composite data types are built from primitive data types. They can hold multiple values or a collection of items. Here are some common composite data types:
Data Type | Description | Example |
---|---|---|
Array | Collection of elements of the same type | int[] numbers = {1, 2, 3}; |
String | Sequence of characters, treated as a single data type | String name = "John"; |
List | Ordered collection that can grow in size | List<String> names = new ArrayList<>(); |
Map | Collection of key-value pairs | Map<String, Integer> map = new HashMap<>(); |
Struct | User-defined data structure combining multiple data types | struct Point { int x; int y; }; |
Understanding Data Types in Different Programming Languages
Different programming languages have variations in data types and their implementations. Let’s take a closer look at how some popular languages define and use data types.
1. Python
Python is dynamically typed, meaning you don’t need to declare a variable’s type explicitly. The interpreter infers the type at runtime.
Data Type | Example |
---|---|
Integer | x = 10 |
Float | y = 5.5 |
String | name = "Alice" |
Boolean | is_active = True |
2. Java
Java is statically typed, requiring explicit data type declarations. This enhances type safety but requires more code.
Data Type | Example |
---|---|
Integer | int x = 10; |
Float | float y = 5.5f; |
String | String name = "Alice"; |
Boolean | boolean isActive = true; |
3. C++
C++ is similar to Java but includes more data types and allows for more complex structures.
Data Type | Example |
---|---|
Integer | int x = 10; |
Float | float y = 5.5; |
Double | double z = 3.14; |
String | std::string name = "Alice"; |
Data Type Conversion
Sometimes, you may need to convert data from one type to another. This process is known as type conversion, and it can be done implicitly or explicitly.
Implicit Conversion
Implicit conversion occurs automatically when the compiler converts one data type to another without any manual intervention.
Example:
int num = 10;
double decimal = num; // Implicit conversion from int to double
Explicit Conversion
Explicit conversion, or type casting, requires the programmer to specify the type conversion.
Example:
double decimal = 5.5;
int num = (int) decimal; // Explicit conversion from double to int
Common Type Conversions
Source Type | Target Type | Description |
---|---|---|
Integer | Float | Implicit conversion possible |
Float | Integer | Requires explicit casting |
String | Integer | Requires parsing |
Character | Integer | Represents ASCII value |
Best Practices for Using Data Types
Understanding data types is not just about knowing their definitions. Here are some best practices to keep in mind:
- Choose the Right Data Type: Selecting the most appropriate data type for your variables can improve performance and readability.
- Be Consistent: Maintain consistency in your data type usage throughout your code to avoid confusion.
- Leverage Strong Typing: Use strongly typed languages where applicable to benefit from type checking at compile time.
- Avoid Magic Numbers: Instead of hardcoding values, use named constants to improve code clarity.
- Utilize Built-in Functions: Familiarize yourself with built-in functions for converting between data types to prevent errors.
Conclusion
Data types are foundational concepts in programming that dictate how data is stored, manipulated, and interpreted. By understanding the different types—primitive and composite—you can make informed decisions about your variable declarations and data management strategies. This knowledge is essential not only for programming but also for effective software testing and development.
As you continue your journey in programming and data structures, remember these principles and best practices. They will enhance your coding skills and ensure high-quality software development.
FAQs
1. What is a data type in programming? A data type is a classification. It specifies the type of data a variable can hold. Examples include integers, floats, or strings.
2. Why are data types important? Data types are important because they dictate how data is stored in memory, affect performance, and help prevent errors.
3. What is the difference between primitive and composite data types? Primitive data types are basic types provided by the language (e.g., integers, floats), while composite data types are built from primitive types and can hold multiple values (e.g., arrays, lists).
4. Can data types be converted? Yes, data types can be converted using implicit or explicit conversion methods, depending on the programming language.
5. How do I choose the right data type? Choose the data type based on the nature of the data you need to store. Consider the operations you will perform. Also, think about the memory requirements.
With this comprehensive guide on data types, you are now equipped to navigate the complexities of programming with confidence. Embrace the power of data types to optimize your coding practices and enhance your software testing capabilities!
Subscribe to QABash Weekly 💥
Dominate – Stay Ahead of 99% Testers!