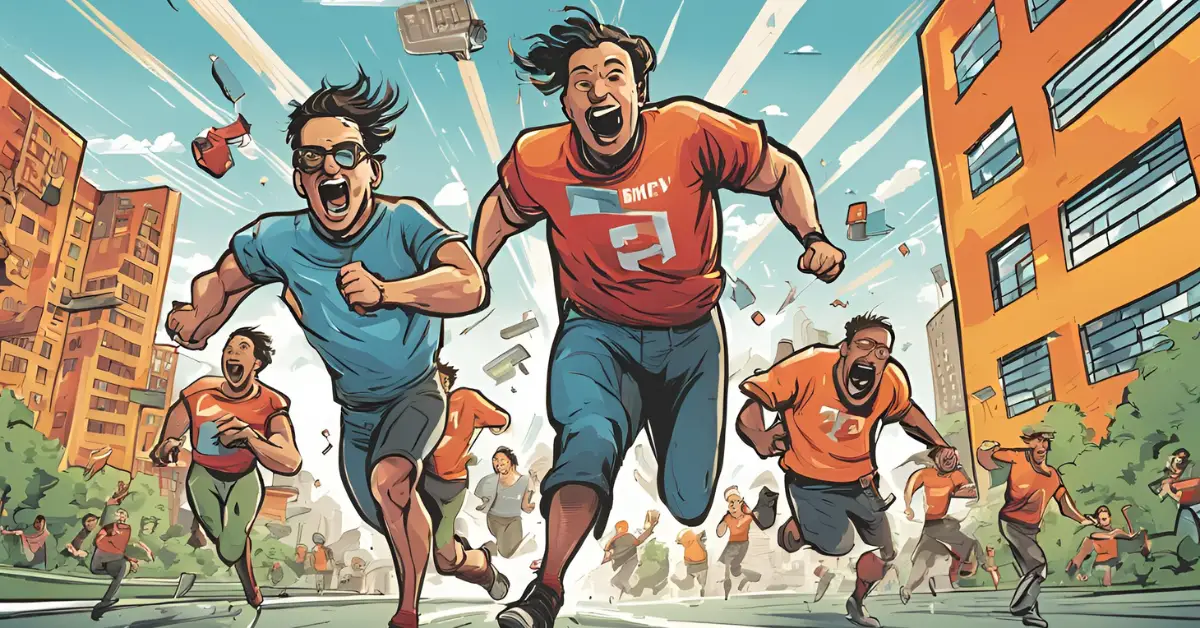
Algorithms are the backbone of computer science and programming. They are step-by-step procedures for solving problems or performing tasks. Understanding how algorithms work and their efficiency is crucial for developers, especially when handling large datasets or optimizing applications. One essential aspect of this understanding is runtime analysis. It allows us to evaluate the performance of algorithms based on their time and space requirements.
In this article, we’ll explore the significance of runtime analysis. We will cover the concept of growth rates and commonly used growth rates. Additionally, we will discuss various types of analysis, notations, and the divide-and-conquer strategy. And, we will cover more. By the end, you’ll have a solid grasp of how to evaluate and compare algorithms effectively.
Why Run Time Analysis?
Runtime analysis helps developers understand how an algorithm performs relative to the size of the input data. This understanding is critical for several reasons:
- Performance Optimization: Identifying which algorithms are faster allows for better performance tuning.
- Resource Management: Knowing the space and time complexity helps in managing system resources efficiently.
- Scalability: Understanding how an algorithm’s performance changes with input size is vital for applications expected to handle large datasets.
- Decision Making: Runtime analysis informs developers about the most suitable algorithm for specific tasks.
Analogy: Choosing the Right Tool
Think of runtime analysis like choosing the right tool for a job. If you’re cutting wood, a chainsaw is faster, but a handsaw be more precise for smaller tasks. Similarly, runtime analysis helps you choose the most effective algorithm based on your performance needs.
Rate of Growth
The rate of growth describes how the runtime of an algorithm increases as the input size grows. This is crucial for understanding the efficiency of algorithms.
Commonly Used Rates of Growth
Here are some common rates of growth and their meanings:
Growth Rate | Notation | Meaning |
---|---|---|
Constant Time | O(1) | The algorithm’s runtime does not change with input size. |
Logarithmic Time | O(log n) | The runtime increases logarithmically as input size increases, often seen in binary search. |
Linear Time | O(n) | The runtime increases linearly with the input size. |
Linearithmic Time | O(n log n) | Common in efficient sorting algorithms like mergesort and heapsort. |
Quadratic Time | O(n²) | The runtime increases quadratically; common in algorithms with nested loops. |
Cubic Time | O(n³) | The runtime increases cubically; often seen in algorithms with three nested loops. |
Exponential Time | O(2^n) | The runtime doubles with each additional element; typically seen in brute-force algorithms. |
Factorial Time | O(n!) | The runtime grows factorially; common in problems that require generating all permutations. |
Types of Analysis
There are several types of analysis used to evaluate algorithms:
1. Worst-case Analysis
This analysis examines the maximum time required for an algorithm to complete. It ensures that even the least efficient cases are accounted for. It provides an upper bound on runtime.
2. Best-case Analysis
Best-case analysis looks at the minimum time an algorithm could take, usually under optimal conditions. While informative, it’s less frequently used for practical performance comparisons.
3. Average-case Analysis
This type of analysis computes the expected runtime over all possible inputs. It often requires assumptions about the distribution of inputs.
4. Amortized Analysis
Amortized analysis averages the time required over a sequence of operations. It provides a more nuanced view of performance over multiple calls rather than a single operation.
5. Guessing and Confirmation
In some algorithms, especially in recursive or complex scenarios, developers may use a technique called “guessing.” This helps estimate the time complexity based on input size and structure. This is often followed by “confirmation” through mathematical proofs or empirical testing to validate these guesses.
Analysis Summary in a Nutshell
Analysis Type | Description |
---|---|
Worst-case | Maximum runtime for the least efficient scenario. |
Best-case | Minimum runtime for the most optimal scenario. |
Average-case | Expected runtime across all possible inputs. |
Amortized | Average time over a series of operations. |
Guessing | Estimating runtime based on input characteristics. |
Confirmation | Validating guesses through proofs or empirical analysis. |
Asymptotic Notation
Asymptotic notation is a mathematical tool used to describe the behavior of functions as the input size approaches infinity. It helps in classifying algorithms based on their runtime and space requirements.
Common Asymptotic Notations
- Big O Notation (O): Describes an upper bound on the runtime, focusing on the worst-case scenario. It provides a limit on how fast an algorithm can go concerning its input size.
- Omega Notation (Ω): Represents the lower bound of an algorithm’s runtime, indicating the best-case scenario. It helps to establish a minimum performance threshold.
- Theta Notation (Θ): Provides a tight bound on the runtime. This means the algorithm’s runtime grows at the same rate for both upper and lower limits. It is useful for characterizing algorithms with predictable performance.
Notations Summary
Notation | Meaning |
---|---|
O(n) | Upper bound on runtime (worst case). |
Ω(n) | Lower bound on runtime (best case). |
Θ(n) | Tight bound on runtime (exactly characterizes performance). |
Divide and Conquer
Divide and conquer is a powerful algorithm design paradigm. It is used to solve complex problems by breaking them down into simpler subproblems. Each subproblem is solved independently, and the solutions are then combined to form a solution to the original problem.
Steps in Divide and Conquer
- Divide: Split the problem into smaller subproblems of the same type.
- Conquer: Solve the subproblems recursively.
- Combine: Merge the solutions of the subproblems to get the final solution.
Example: Merge Sort
Merge Sort is a classic example of a divide-and-conquer algorithm.
public void mergeSort(int[] array) {
if (array.length < 2) return;
int mid = array.length / 2;
int[] left = Arrays.copyOfRange(array, 0, mid);
int[] right = Arrays.copyOfRange(array, mid, array.length);
mergeSort(left);
mergeSort(right);
merge(array, left, right);
}
private void merge(int[] array, int[] left, int[] right) {
int i = 0, j = 0, k = 0;
while (i < left.length && j < right.length) {
if (left[i] <= right[j]) {
array[k++] = left[i++];
} else {
array[k++] = right[j++];
}
}
while (i < left.length) {
array[k++] = left[i++];
}
while (j < right.length) {
array[k++] = right[j++];
}
}
Runtime Analysis of Merge Sort
- Worst-case: O(n log n) – The array is divided log n times, and merging takes linear time.
- Best-case: O(n log n) – Even in the best-case scenario, merging is still required.
- Average-case: O(n log n) – The expected time remains consistent across different inputs.
Conclusion
Understanding runtime analysis, growth rates, asymptotic notations, and the divide-and-conquer strategy is essential. These concepts are crucial for developing efficient algorithms and optimizing software performance. By mastering these concepts, you empower yourself to make informed decisions about algorithm selection and performance optimization.
As you delve deeper into algorithms, keep in mind the different types of analysis and notations. They will be invaluable tools in your programming toolkit. Knowing how to evaluate and compare algorithms will enhance your coding skills. It will also improve the efficiency of your applications.
FAQs
1. What is runtime analysis?
Runtime analysis is the evaluation of an algorithm’s performance based on time and space requirements as the input size grows.
2. Why are growth rates important?
Growth rates help in understanding how the runtime of an algorithm changes with input size, allowing for better performance predictions.
3. What are the common types of analysis for algorithms?
Common types include worst-case, best-case, average-case, amortized analysis, guessing, and confirmation.
4. What do Big O, Omega, and Theta notations represent?
Big O denotes an upper bound, Omega denotes a lower bound, and Theta provides a tight bound on runtime.
5. How can I determine which algorithm to use?
Consider the size of the input, the required efficiency, and the specific needs of your application when choosing an algorithm.
6. What is the divide-and-conquer strategy?
Divide-and-conquer is an algorithm design paradigm that breaks a problem into smaller subproblems, solves them independently, and combines their solutions.
With these insights, you’re now better equipped to navigate the fascinating world of algorithms and their performance analysis!
Subscribe to QABash Weekly 
Dominate – Stay Ahead of 99% Testers!