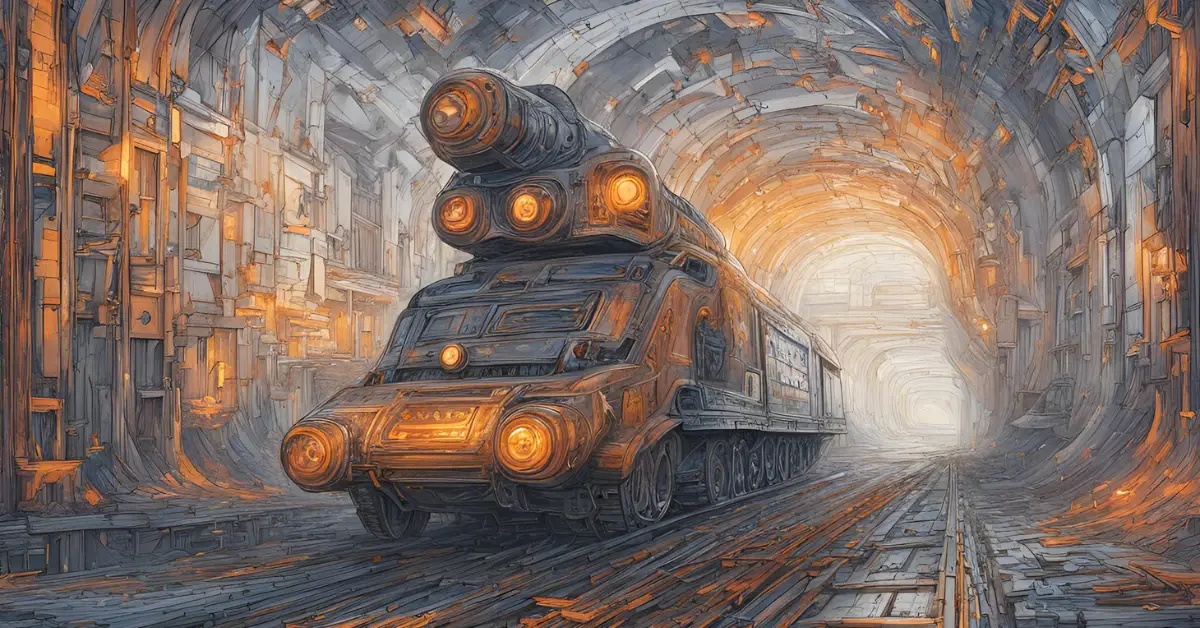
Introduction
TensorFlow is an open-source machine learning framework developed by Google. It is widely used for building and deploying machine learning models. This tutorial will guide you through the basics of TensorFlow, from installation to creating and training your first neural network.
Key Concepts of TensorFlow
- Tensors: Multi-dimensional arrays that are the core data structures in TensorFlow.
- Graphs: Define the computations to be performed.
- Sessions: Execute the operations defined in the graphs.
- Variables: Hold and update parameters during training.
- Placeholders: Used to feed data into the model.
Installing TensorFlow
Using pip
pip install tensorflow
Using Conda
conda create -n tf_env tensorflow conda activate tf_env
Basic Operations with Tensors
Creating Tensors
import tensorflow as tf # Creating constant tensors tensor1 = tf.constant(3.0, dtype=tf.float32) tensor2 = tf.constant(4.0) # Creating tensors with specific values tensor3 = tf.zeros([3, 3]) tensor4 = tf.ones([2, 3]) tensor5 = tf.random.normal([2, 2], mean=0.0, stddev=1.0) print(tensor1, tensor2) print(tensor3) print(tensor4) print(tensor5)
Basic Operations
codea = tf.constant([[1, 2], [3, 4]]) b = tf.constant([[5, 6], [7, 8]]) # Addition result_add = tf.add(a, b) # Multiplication result_mul = tf.matmul(a, b) print("Addition:\n", result_add.numpy()) print("Multiplication:\n", result_mul.numpy())
Building and Training a Neural Network
Loading and Preprocessing Data
from tensorflow.keras.datasets import mnist # Load MNIST dataset (x_train, y_train), (x_test, y_test) = mnist.load_data() # Normalize the data x_train, x_test = x_train / 255.0, x_test / 255.0 # Reshape the data x_train = x_train.reshape(-1, 28, 28, 1) x_test = x_test.reshape(-1, 28, 28, 1) print(f"Training data shape: {x_train.shape}") print(f"Test data shape: {x_test.shape}")
Creating the Model
from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense, Flatten, Conv2D, MaxPooling2D # Building the model model = Sequential([ Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)), MaxPooling2D(pool_size=(2, 2)), Flatten(), Dense(128, activation='relu'), Dense(10, activation='softmax') ]) # Compile the model model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
Training the Model
# Train the model model.fit(x_train, y_train, epochs=5, validation_split=0.2)
Evaluating the Model
# Evaluate the model test_loss, test_acc = model.evaluate(x_test, y_test, verbose=2) print(f"Test accuracy: {test_acc}")
Making Predictions
# Making predictions predictions = model.predict(x_test) # Displaying the first prediction import numpy as np print("Predicted label:", np.argmax(predictions[0])) print("True label:", y_test[0])
Saving and Loading the Model
Saving the Model
# Save the entire model model.save('my_model.h5')
Loading the Model
# Load the model new_model = tf.keras.models.load_model('my_model.h5') # Check its architecture new_model.summary()
Best Practices for TensorFlow
- Use tf.data for Data Pipelines
- Use the
tf.data
API to build efficient input pipelines.
- Use the
- Use TensorBoard for Monitoring
- Use TensorBoard to visualize metrics such as loss and accuracy during training.
- Leverage Pre-trained Models
- Use pre-trained models from TensorFlow Hub or Keras Applications to save time and resources.
- Regularization Techniques
- Apply regularization techniques like dropout and batch normalization to prevent overfitting.
- Optimize Model Performance
- Optimize model performance using techniques like mixed precision training and model quantization.
Conclusion
Getting started with TensorFlow involves understanding its key concepts, setting up the environment, and performing basic operations with tensors. Building, training, and evaluating a neural network using TensorFlow is straightforward with the high-level Keras API. By following best practices, you can create efficient and scalable machine learning models. Regularly saving and loading models ensures that your progress is preserved and models can be reused or shared easily.
Subscribe to QABash Weekly 💥
Dominate – Stay Ahead of 99% Testers!