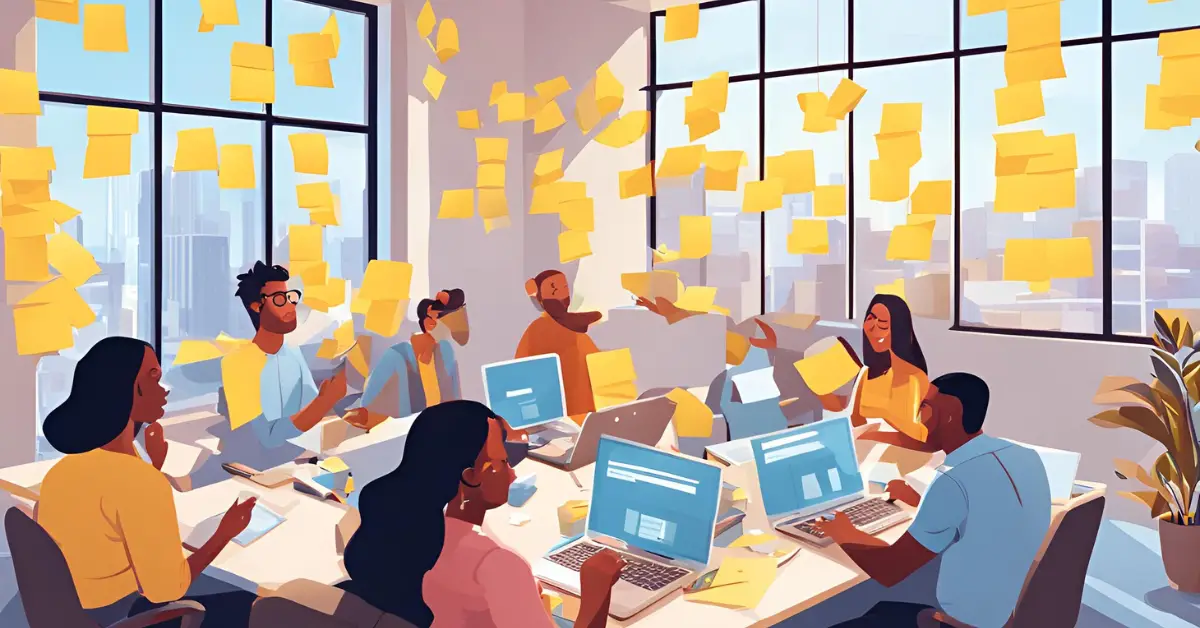
General Playwright Questions
- What is Playwright?
- Answer: Playwright is an open-source framework developed by Microsoft for automating web browsers. It allows you to write scripts to test and interact with web applications across different browsers like Chromium, Firefox, and WebKit.
- How does Playwright differ from Selenium?
- Answer: While both are used for browser automation, Playwright is designed to be more modern and robust with native support for multiple browsers and parallel testing. It provides a more consistent API and handles asynchronous operations more effectively.
- What are the core features of Playwright?
- Answer: Core features include cross-browser support (Chromium, Firefox, WebKit), automatic waiting, network interception, screenshots and video recording, and built-in test runner.
- How do you install Playwright?
- Answer: You can install Playwright using npm with the command
npm install playwright
. To install Playwright along with its dependencies, you can usenpx playwright install
.
- Answer: You can install Playwright using npm with the command
- What browsers are supported by Playwright?
- Answer: Playwright supports Chromium (including Google Chrome and Microsoft Edge), Firefox, and WebKit (Safari).
- What is a browser context in Playwright?
- Answer: A browser context is an isolated environment in which you can create multiple browser instances. Each context has its own cookies, local storage, and session data, allowing you to test different scenarios in parallel.
- How do you launch a browser in Playwright?
- Answer: You can launch a browser using
playwright.chromium.launch()
,playwright.firefox.launch()
, orplaywright.webkit.launch()
depending on the browser you want to use.
- Answer: You can launch a browser using
- What is the purpose of
page.goto()
in Playwright?- Answer:
page.goto()
navigates the browser to a specified URL, allowing you to load a web page and interact with its content.
- Answer:
- How does Playwright handle asynchronous operations?
- Answer: Playwright uses JavaScriptโs async/await syntax to handle asynchronous operations, ensuring that actions like page loads and element interactions are completed before moving to the next step.
- How can you take a screenshot in Playwright?
- Answer: You can take a screenshot by calling
page.screenshot({ path: 'screenshot.png' })
to save a screenshot of the current page.
- Answer: You can take a screenshot by calling
Test Automation
- How do you write a simple test using Playwright?
- Answer: A simple test can be written as follows:
const { test, expect } = require('@playwright/test');
test('simple test', async ({ page }) => { await page.goto('https://example.com');
const title = await page.title();
expect(title).toBe('Example Domain'); });
- What is the role of the
expect
function in Playwright?- Answer: The
expect
function is used for assertions in tests. It allows you to verify that certain conditions are met, such as element visibility or text content.
- Answer: The
- How do you handle authentication in Playwright tests?
- Answer: Authentication can be handled by either using cookies or by programmatically logging in via page interactions. You can save the authentication state and reuse it across tests using
context.storageState()
.
- Answer: Authentication can be handled by either using cookies or by programmatically logging in via page interactions. You can save the authentication state and reuse it across tests using
- How can you wait for an element to be visible in Playwright?
- Answer: You can use
await page.waitForSelector('selector', { state: 'visible' })
to wait until an element becomes visible.
- Answer: You can use
- What is Playwright Test Runner?
- Answer: Playwright Test Runner is a built-in test framework that simplifies writing and running tests. It supports features like parallel test execution, retries, and test reporting.
- How do you handle dynamic content in Playwright?
- Answer: Use waiting strategies such as
waitForSelector
,waitForFunction
, orwaitForResponse
to handle dynamic content that loads asynchronously.
- Answer: Use waiting strategies such as
- How can you interact with forms in Playwright?
- Answer: You can interact with forms using methods like
fill()
,check()
,uncheck()
, andselectOption()
to fill out input fields, select checkboxes, and choose options from dropdowns.
- Answer: You can interact with forms using methods like
- How do you mock network requests in Playwright?
- Answer: You can mock network requests by using
page.route()
to intercept and modify network requests or responses.
- Answer: You can mock network requests by using
- What is
page.evaluate()
used for?- Answer:
page.evaluate()
allows you to execute JavaScript code within the context of the page, which is useful for interacting with the pageโs DOM or executing custom scripts.
- Answer:
- How do you handle browser popups in Playwright?
- Answer: You can handle popups by listening for
page.on('dialog')
and then responding to the dialog using methods likedialog.accept()
ordialog.dismiss()
.
- Answer: You can handle popups by listening for
Advanced Topics
- What is
browser.newContext()
used for?- Answer:
browser.newContext()
creates a new browser context, which is useful for testing different user sessions or scenarios in isolation.
- Answer:
- How do you run tests in parallel using Playwright?
- Answer: You can run tests in parallel by using the Playwright Test Runner, which handles parallel execution by default. You can configure parallelism using the
workers
option in the test configuration.
- Answer: You can run tests in parallel by using the Playwright Test Runner, which handles parallel execution by default. You can configure parallelism using the
- How can you take a full-page screenshot in Playwright?
- Answer: Use
page.screenshot({ path: 'fullpage.png', fullPage: true })
to capture a full-page screenshot, including parts of the page that are not visible in the viewport.
- Answer: Use
- How do you record videos of your tests in Playwright?
- Answer: You can record videos by setting the
recordVideo
option when creating a new context:const context = await browser.newContext({ recordVideo: { dir: 'videos/' } });
.
- Answer: You can record videos by setting the
- What is Playwrightโs tracing feature?
- Answer: Tracing in Playwright allows you to record detailed information about test execution, including screenshots, network requests, and DOM snapshots, which is useful for debugging.
- How can you manage browser permissions in Playwright?
- Answer: Use
context.grantPermissions()
to grant specific permissions, such as geolocation or notifications, to the browser context.
- Answer: Use
- How do you handle iframes in Playwright?
- Answer: Access iframes using the
frame
method on the page object. For example,const frame = page.frame({ name: 'frameName' });
.
- Answer: Access iframes using the
- What are Playwrightโs built-in assertions?
- Answer: Playwrightโs built-in assertions include methods like
toBe()
,toEqual()
,toContain()
,toHaveText()
, and more, which help in verifying the state of elements or values.
- Answer: Playwrightโs built-in assertions include methods like
- How do you manage test data and fixtures in Playwright?
- Answer: Use fixtures in the Playwright Test Runner to set up and tear down test data. Fixtures can be defined to provide reusable test data or context setup.
- How do you use custom test reporters in Playwright?
- Answer: You can implement a custom test reporter by extending the
Reporter
class and configuring it in the Playwright configuration file under thereporter
option.
- Answer: You can implement a custom test reporter by extending the
Troubleshooting and Optimization
- How can you debug Playwright tests?
- Answer: Use Playwrightโs built-in debugging tools like the
DEBUG
environment variable,page.pause()
, andtrace
feature. You can also use Playwright Inspector for interactive debugging.
- Answer: Use Playwrightโs built-in debugging tools like the
- What is the purpose of the
page.pause()
method?- Answer:
page.pause()
pauses the test execution and opens Playwright Inspector, allowing you to inspect the state of the page and interact with it manually.
- Answer:
- How do you handle flaky tests in Playwright?
- Answer: Identify the root cause of flakiness, such as timing issues or network instability, and use strategies like retries or more robust waiting mechanisms to handle them.
- How can you optimize the performance of Playwright tests?
- Answer: Optimize performance by running tests in parallel, using headless mode, reducing unnecessary waits, and leveraging efficient locators.
- What strategies can be used to handle network latency in Playwright tests?
- Answer: Use network interception to mock or stub responses, employ retries for flaky network requests, and use appropriate wait strategies.
- How do you handle JavaScript errors on the page during testing?
- Answer: Listen for
page.on('pageerror')
to capture JavaScript errors that occur during test execution.
- Answer: Listen for
- What are some common issues encountered with Playwright tests?
- Answer: Common issues include timing problems, unstable locators, and differences between browsers. Address these by using robust locators, improving waiting strategies, and using browser-specific options if needed.
- How do you manage browser versions in Playwright?
- Answer: Playwright automatically manages browser binaries, but you can specify versions or install additional browsers using
playwright install
.
- Answer: Playwright automatically manages browser binaries, but you can specify versions or install additional browsers using
Troubleshooting and Optimization (continued)
- What is the role of the
browser.close()
method?
- Answer:
browser.close()
shuts down the browser instance and frees up system resources, which helps avoid memory leaks and ensures that each test starts with a fresh browser state.
- How do you handle slow network responses in Playwright tests?
- Answer: You can handle slow network responses by using
page.waitForResponse()
with a timeout, or by using theroute
method to mock slow responses to avoid delays in your tests.
- How do you manage dependencies and setup for Playwright tests?
- Answer: Use test fixtures and setup files to manage dependencies and setup. Define common setup steps in a
globalSetup
orglobalTeardown
script and use fixtures to provide reusable test data.
- What is
page.waitForFunction()
used for?
- Answer:
page.waitForFunction()
waits for a specific condition to be true in the page context, which is useful for waiting for dynamic content or custom conditions.
- How can you use Playwright with CI/CD pipelines?
- Answer: Integrate Playwright with CI/CD pipelines by configuring your pipeline to install dependencies, run Playwright tests, and handle reports and artifacts. Most CI/CD systems support running Playwright tests in their environments.
- How do you ensure cross-browser compatibility with Playwright?
- Answer: Write tests that are designed to be cross-browser compatible and run those tests across different browsers supported by Playwright. Utilize browser-specific features or configurations if necessary.
- What are Playwrightโs capabilities for handling file uploads?
- Answer: You can handle file uploads using the
setInputFiles()
method on file input elements, which allows you to simulate file selection during tests.
- How do you deal with browser caching issues in Playwright?
- Answer: You can clear the browser cache by creating a new browser context or using
context.clearCookies()
to remove cookies that might affect the test.
- How can you manage user sessions in Playwright?
- Answer: Save and reuse user sessions by storing the authentication state with
context.storageState()
and restoring it for subsequent tests.
- What strategies can be used to handle browser timeouts in Playwright?
- Answer: Increase the timeout values using options like
timeout
in methods likepage.goto()
orwaitForSelector()
. Also, consider usingpage.waitForFunction()
to handle specific conditions that might require longer wait times.
- How do you deal with CAPTCHA challenges in automated tests?
- Answer: Automated tests should ideally avoid CAPTCHA challenges by using test-specific accounts or environments where CAPTCHA is disabled. If thatโs not possible, you might need to use CAPTCHA-solving services or manual intervention.
- What are some best practices for writing Playwright tests?
- Answer: Some best practices include:
- Write clear, maintainable tests with descriptive names.
- Use robust selectors to avoid test fragility.
- Implement proper error handling and retries for flaky tests.
- Leverage fixtures and configuration to manage test data and setup.
- Use parallel execution to speed up test runs.
- Regularly update dependencies and Playwright versions to benefit from the latest features and fixes.
Subscribe to QABash Weekly ๐ฅ
Dominate โ Stay Ahead of 99% Testers!