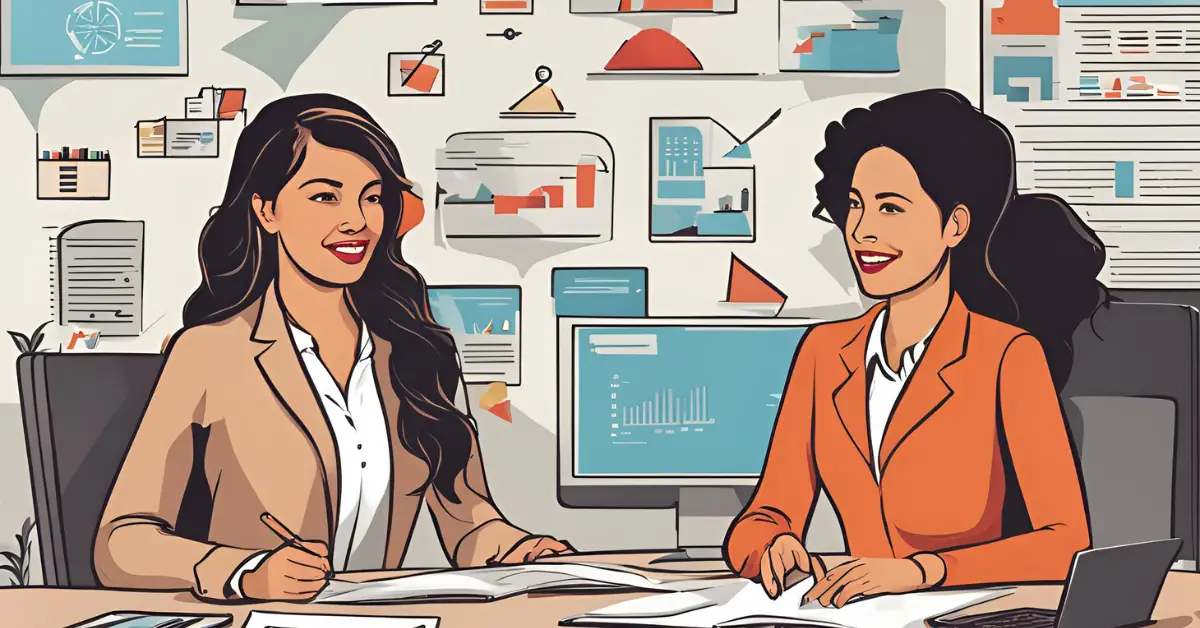
General Cypress Questions
- What is Cypress?
- Answer: Cypress is an open-source end-to-end testing framework designed for modern web applications. It enables developers to write tests that interact with the user interface and verify the applicationโs functionality.
- How does Cypress differ from Selenium?
- Answer: Unlike Selenium, which operates by interacting with the browser through WebDriver, Cypress runs inside the browser itself. This provides more reliable and faster test execution, automatic waiting, and easier debugging.
- What are the core features of Cypress?
- Answer: Core features include real-time browser preview, automatic waiting, easy setup, time-travel debugging, network stubbing, and support for both unit and integration testing.
- How do you install Cypress?
- Answer: Install Cypress using npm with the command
npm install cypress --save-dev
. You can then open Cypress withnpx cypress open
.
- Answer: Install Cypress using npm with the command
- What is the Cypress Test Runner?
- Answer: The Cypress Test Runner is an interactive UI that allows you to run and debug your tests directly in the browser. It provides real-time feedback and allows you to see the test execution as it happens.
- What is a Cypress test suite?
- Answer: A Cypress test suite is a collection of tests organized together. In Cypress, test suites are created using
describe()
blocks.
- Answer: A Cypress test suite is a collection of tests organized together. In Cypress, test suites are created using
- What is a Cypress test case?
- Answer: A Cypress test case is an individual test within a suite, defined using the
it()
function. It contains the logic for a specific test scenario.
- Answer: A Cypress test case is an individual test within a suite, defined using the
- How do you write a basic test in Cypress?
- Answer: A basic test might look like this:
describe('My First Test', () => {
it('Visits the Cypress website', () => {
cy.visit('https://www.cypress.io');
cy.contains('Get Started').click();
});
});
- Answer: A basic test might look like this:
- What is
cy.visit()
used for in Cypress?- Answer:
cy.visit()
is used to navigate to a specific URL. It loads the page and allows you to interact with it.
- Answer:
- How do you assert values in Cypress tests?
- Answer: Use assertions provided by Cypress commands. For example,
cy.get('selector').should('contain', 'text')
checks if the element contains specific text.
- Answer: Use assertions provided by Cypress commands. For example,
Cypress Commands and Assertions
- What is
cy.get()
used for?- Answer:
cy.get()
is used to select elements in the DOM based on a CSS selector. It is a core command for querying elements.
- Answer:
- How do you interact with form elements in Cypress?
- Answer: Use commands like
cy.type()
,cy.select()
,cy.check()
, andcy.uncheck()
to interact with input fields, dropdowns, and checkboxes.
- Answer: Use commands like
- What is
cy.contains()
used for?- Answer:
cy.contains()
is used to find an element that contains specific text, which is useful for locating elements based on their displayed content.
- Answer:
- How do you handle asynchronous operations in Cypress?
- Answer: Cypress automatically waits for commands and assertions to complete. You do not need to use explicit waits or callbacks as Cypress handles asynchronous operations internally.
- What is
cy.wait()
used for?- Answer:
cy.wait()
is used to wait for a specific period or for an XHR request to complete. It helps in scenarios where you need to wait for some operation to finish before proceeding.
- Answer:
- How can you stub network requests in Cypress?
- Answer: Use
cy.intercept()
to stub or modify network requests. This allows you to simulate responses and control the behavior of your application during tests.
- Answer: Use
- What is the purpose of
cy.fixture()
?- Answer:
cy.fixture()
is used to load external files, such as JSON or text files, for use in tests. It helps in providing test data or mocking responses.
- Answer:
- How do you handle file uploads in Cypress?
- Answer: Use the
cy.upload()
plugin or simulate file uploads by setting thefiles
input property using JavaScript.
- Answer: Use the
- What is
cy.screenshot()
used for?- Answer:
cy.screenshot()
is used to take screenshots of the current state of the application during a test, which is useful for debugging and visual validation.
- Answer:
- How do you use custom commands in Cypress?
- Answer: Define custom commands in
cypress/support/commands.js
usingCypress.Commands.add()
. Custom commands help in reusing code and simplifying test scripts.
- Answer: Define custom commands in
Advanced Topics
- What is a Cypress fixture?
- Answer: Fixtures are static files used to provide data to tests. They are stored in the
cypress/fixtures
folder and can be accessed usingcy.fixture()
.
- Answer: Fixtures are static files used to provide data to tests. They are stored in the
- How do you manage environment variables in Cypress?
- Answer: Manage environment variables using the
cypress.json
configuration file or by setting them directly in your test commands. They can be accessed usingCypress.env()
.
- Answer: Manage environment variables using the
- What is the role of
cypress.json
?- Answer:
cypress.json
is the configuration file for Cypress where you can define various settings such as base URL, environment variables, and default timeouts.
- Answer:
- How do you run Cypress tests in headless mode?
- Answer: Run Cypress tests in headless mode by using the command
npx cypress run
. This executes the tests without opening the Test Runner UI.
- Answer: Run Cypress tests in headless mode by using the command
- What is
cy.exec()
used for?- Answer:
cy.exec()
is used to execute system commands or scripts as part of the test process, allowing you to perform tasks like setting up or cleaning up the test environment.
- Answer:
- How do you implement data-driven testing in Cypress?
- Answer: Implement data-driven testing by using fixtures or external data sources and iterating over data in your tests. You can also use
cy.each()
to iterate through data sets.
- Answer: Implement data-driven testing by using fixtures or external data sources and iterating over data in your tests. You can also use
- What is the purpose of the
cypress/plugins/index.js
file?- Answer: The
cypress/plugins/index.js
file is used to configure plugins and extend Cypress functionality. It is where you can define custom behavior or integrations.
- Answer: The
- How do you use Cypress with CI/CD pipelines?
- Answer: Integrate Cypress with CI/CD pipelines by configuring your pipeline scripts to install Cypress, run tests, and handle results. Most CI/CD tools have plugins or support for Cypress.
- What are Cypress โbeforeโ and โafterโ hooks?
- Answer: โbeforeโ and โafterโ hooks are used to run code before or after each test or test suite.
before()
runs before tests, andafter()
runs after tests. These hooks are useful for setup and teardown operations.
- Answer: โbeforeโ and โafterโ hooks are used to run code before or after each test or test suite.
- What is the
cy.request()
command used for?- Answer:
cy.request()
is used to make HTTP requests to an API endpoint directly, allowing you to test API responses and perform actions like authentication or data setup.
- Answer:
Troubleshooting and Debugging
- How do you debug Cypress tests?
- Answer: Use the Cypress Test Runner for interactive debugging, utilize
cy.debug()
to pause execution, inspect elements using browser dev tools, and usecy.screenshot()
for visual feedback.
- Answer: Use the Cypress Test Runner for interactive debugging, utilize
- What is the role of
cy.pause()
?- Answer:
cy.pause()
is used to pause the test execution and allow manual interaction with the application during testing. It helps in debugging issues interactively.
- Answer:
- How do you handle flaky tests in Cypress?
- Answer: Address flaky tests by improving test reliability, using proper waits, handling dynamic content, and avoiding hardcoded timeouts. Review logs and debug to identify root causes.
- What is
cy.visit({ timeout: ... })
used for?- Answer:
cy.visit({ timeout: ... })
allows you to specify a timeout for page load, which is useful for handling slow-loading pages and preventing tests from failing due to extended load times.
- Answer:
- How do you use Cypress to test responsive design?
- Answer: Test responsive design by using the
cy.viewport()
command to simulate different screen sizes and devices, and verify that the application behaves correctly across various viewports.
- Answer: Test responsive design by using the
- How do you handle browser compatibility in Cypress?
- Answer: Cypress supports testing in Chrome, Firefox, and Edge. You can configure your tests to run in different browsers and ensure cross-browser compatibility by using the appropriate test settings and configurations.
- How do you manage test data and state in Cypress?
- Answer: Manage test data and state by using fixtures, stubbing network requests, and setting up application state before tests. You can also use
cy.task()
to perform backend operations.
- Answer: Manage test data and state by using fixtures, stubbing network requests, and setting up application state before tests. You can also use
- What are some best practices for writing Cypress tests?
- Answer: Best practices include writing clear and maintainable tests, using meaningful test names, avoiding hardcoded values, using fixtures for test data, and ensuring tests are isolated and independent.
- How do you handle authentication in Cypress tests?
- Answer: Handle authentication by stubbing authentication requests using
cy.intercept()
or by logging in programmatically usingcy.request()
to set authentication tokens or cookies.
- Answer: Handle authentication by stubbing authentication requests using
- How do you use
cy.wrap()
in Cypress?- Answer:
cy.wrap()
is used to wrap non-Cypress objects or values into a Cypress chainable object, allowing you to perform Cypress commands or assertions on them.
- Answer:
Advanced Topics and Integration
- What is the purpose of Cypress plugins?
- Answer: Cypress plugins extend the functionality of Cypress by providing additional features, integrating with other tools, or customizing test execution. They are configured in
cypress/plugins/index.js
.
- Answer: Cypress plugins extend the functionality of Cypress by providing additional features, integrating with other tools, or customizing test execution. They are configured in
- How do you create a custom Cypress plugin?
- Answer: Create a custom plugin by defining it in
cypress/plugins/index.js
and implementing the desired functionality. Plugins can be used to add custom commands, modify test behavior, or integrate with external tools.
- Answer: Create a custom plugin by defining it in
- What is
cy.task()
used for in Cypress?- Answer:
cy.task()
is used to run Node.js code from within a Cypress test. It allows you to perform tasks such as interacting with the file system, connecting to databases, or executing custom scripts.
- Answer:
- How do you configure global test settings in Cypress?
- Answer: Configure global test settings in
cypress.json
, where you can define base URL, timeouts, environment variables, and other global configuration options.
- Answer: Configure global test settings in
- What is the purpose of Cypressโ
cypress.json
file?- Answer: The
cypress.json
file is used for global configuration settings for Cypress tests. It includes base URL, viewport settings, environment variables, and other configuration options.
- Answer: The
- How do you use Cypress for visual regression testing?
- Answer: Use visual regression plugins like
cypress-image-snapshot
to compare screenshots of your applicationโs UI with baseline images and detect visual changes.
- Answer: Use visual regression plugins like
- What is Cypress Dashboard?
- Answer: Cypress Dashboard is a service provided by Cypress that offers advanced features such as test recording, parallelization, and detailed test analytics. It helps in tracking and managing test runs.
- How do you implement parallel test execution in Cypress?
- Answer: Implement parallel test execution by using the Cypress Dashboard service or CI/CD tools that support parallel test execution. Configure your CI/CD pipeline to distribute test runs across multiple agents.
- What is the use of the
cy.wrap()
command?- Answer:
cy.wrap()
allows you to convert a non-Cypress object into a Cypress chainable object, enabling you to use Cypress commands and assertions on it.
- Answer:
- How do you handle cookies in Cypress?
- Answer: Handle cookies using commands like
cy.getCookie()
,cy.setCookie()
, andcy.clearCookie()
. These commands allow you to interact with cookies for tasks such as setting authentication tokens or clearing session data.
- Answer: Handle cookies using commands like
Subscribe to QABash Weekly ๐ฅ
Dominate โ Stay Ahead of 99% Testers!