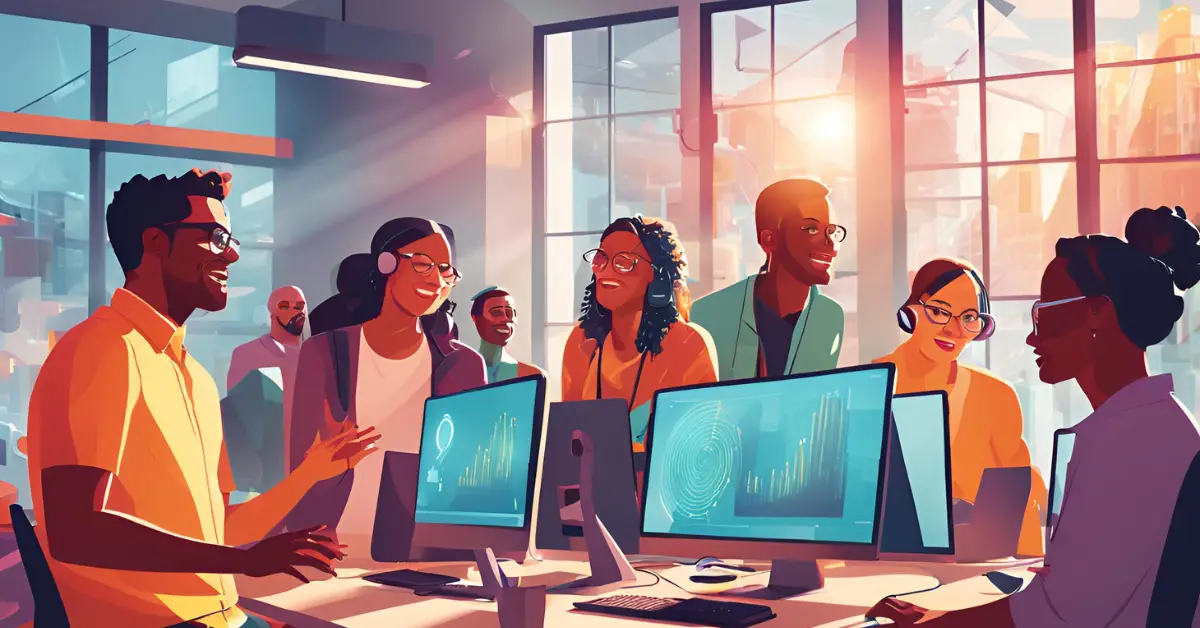
1. What is Selenium?
Answer: Selenium is an open-source suite of tools used for automating web browsers. It supports multiple programming languages like Java, C#, Python, and more. Selenium is widely used for web application testing.
2. What are the different components of Selenium?
Answer: The main components of Selenium are:
- Selenium WebDriver: Provides a programming interface to create and execute test scripts.
- Selenium IDE: A browser extension that allows recording and playback of test scripts.
- Selenium Grid: Allows parallel execution of tests across different machines and browsers.
- Selenium RC (Remote Control): An older component used for running tests on a browser remotely.
3. What is Selenium WebDriver?
Answer: Selenium WebDriver is a component of Selenium that provides a programming interface to interact with and control web browsers. It replaces Selenium RC and is more efficient and easier to use.
4. What is the difference between Selenium WebDriver and Selenium RC?
Answer: Selenium RC uses a server to inject JavaScript code into web pages, which is less efficient and more complex. Selenium WebDriver interacts directly with the browser and provides better performance and simpler API.
5. What is Selenium Grid?
Answer: Selenium Grid is used for parallel execution of tests across multiple machines and browsers. It helps in speeding up the testing process and managing tests on different environments.
6. What is Selenium IDE?
Answer: Selenium IDE (Integrated Development Environment) is a browser extension that allows users to record and playback tests. It is useful for creating simple tests quickly but is limited compared to WebDriver.
7. What programming languages are supported by Selenium?
Answer: Selenium supports multiple programming languages, including Java, C#, Python, Ruby, JavaScript (Node.js), and Kotlin.
8. What is XPath in Selenium?
Answer: XPath (XML Path Language) is used to locate elements on a web page. It provides a way to navigate through elements and attributes in XML documents. In Selenium, XPath is used to identify web elements for interaction.
9. What is the difference between XPath and CSS Selectors?
Answer: XPath is a language for selecting nodes from XML documents, while CSS Selectors are used to select HTML elements based on their styles. XPath allows more complex queries, whereas CSS Selectors are generally faster.
10. How can you handle alerts in Selenium?
Answer: Alerts can be handled using the Alert
interface in Selenium. You can switch to an alert, accept or dismiss it, and retrieve its text.
Alert alert = driver.switchTo().alert();
alert.accept(); // To accept the alert
alert.dismiss(); // To dismiss the alert
String text = alert.getText(); // To get the alert text
11. How do you handle dropdowns in Selenium?
Answer: Dropdowns can be handled using the Select
class in Selenium. You can select options by visible text, index, or value.
Select dropdown = new Select(driver.findElement(By.id("dropdownId")));
dropdown.selectByVisibleText("Option Text");
dropdown.selectByIndex(1);
dropdown.selectByValue("optionValue");
12. What is a WebDriverWait in Selenium?
Answer: WebDriverWait
is used to wait for a certain condition to occur before proceeding with the execution of the script. It helps in handling dynamic web elements.
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
13. What is the Page Object Model (POM)?
Answer: The Page Object Model is a design pattern in Selenium where each web page is represented by a separate class. This helps in creating maintainable and reusable test code.
14. What is the difference between findElement
and findElements
?
Answer: findElement
returns a single WebElement
that matches the locator, while findElements
returns a list of WebElement
objects that match the locator.
15. How can you perform mouse actions in Selenium?
Answer: Mouse actions can be performed using the Actions
class in Selenium. It allows for operations like clicking, double-clicking, hovering, and drag-and-drop.
Actions actions = new Actions(driver);
actions.moveToElement(element).click().perform();
16. How do you handle iframes in Selenium?
Answer: To handle iframes, you need to switch the driver’s context to the iframe before interacting with elements inside it.
driver.switchTo().frame("frameNameOrId");
driver.findElement(By.id("elementId")).click();
driver.switchTo().defaultContent(); // To switch back to the main content
17. How do you take a screenshot in Selenium?
Answer: You can take a screenshot using the TakesScreenshot
interface in Selenium.
File screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(screenshot, new File("path/to/screenshot.png"));
18. What is the use of JavascriptExecutor
in Selenium?
Answer: JavascriptExecutor
allows you to execute JavaScript code within the context of the browser. It is useful for performing actions that are not directly supported by Selenium.
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("alert('Hello World!');");
19. How can you handle file uploads in Selenium?
Answer: File uploads can be handled by sending the file path directly to the file input element.
driver.findElement(By.id("fileUpload")).sendKeys("path/to/file.txt");
20. How do you handle dynamic elements in Selenium?
Answer: Dynamic elements can be handled by using strategies like WebDriverWait
to wait for the element to be present or visible before interacting with it.
21. How can you switch between multiple windows in Selenium?
Answer: You can switch between multiple windows using the window handles provided by Selenium.
String mainWindow = driver.getWindowHandle();
Set<String> allWindows = driver.getWindowHandles();
for (String window : allWindows) {
if (!window.equals(mainWindow)) {
driver.switchTo().window(window);
}
}
22. How do you perform drag and drop in Selenium?
Answer: Drag and drop actions can be performed using the Actions
class.
Actions actions = new Actions(driver);
actions.dragAndDrop(sourceElement, targetElement).perform();
23. What is the purpose of the Driver.manage().timeouts()
method?
Answer: Driver.manage().timeouts()
is used to set various timeout values, such as page load timeout, implicit wait timeout, and script timeout.
driver.manage().timeouts().pageLoadTimeout(10, TimeUnit.SECONDS);
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
24. How do you execute a JavaScript alert in Selenium?
Answer: JavaScript alerts can be executed using JavascriptExecutor
.
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("alert('This is a JavaScript alert!');");
25. What is a test suite in Selenium?
Answer: A test suite is a collection of test cases that are grouped together. It allows running multiple tests in a specific order and managing their execution efficiently.
26. How do you create a test suite in Selenium?
Answer: Test suites can be created using test frameworks like TestNG or JUnit. In TestNG, you can create an XML file to define test suites and test cases.
27. What are the types of waits in Selenium?
Answer: There are two main types of waits in Selenium:
- Implicit Wait: Automatically applies to all elements and waits for a specified time.
- Explicit Wait: Waits for a specific condition to occur before proceeding.
28. How can you handle pop-ups in Selenium?
Answer: Pop-ups can be handled by switching to the pop-up window using driver.switchTo().window()
, or handling alerts using the Alert
interface.
29. How can you test a file download in Selenium?
Answer: File downloads are typically tested by verifying that the file is downloaded to the specified location and has the correct content.
30. How can you handle different browsers in Selenium?
Answer: Selenium WebDriver supports different browsers by using browser-specific drivers (e.g., ChromeDriver for Chrome, GeckoDriver for Firefox).
31. How can you execute a test case in parallel in Selenium?
Answer: Parallel execution can be achieved using Selenium Grid or test frameworks like TestNG that support parallel test execution.
32. What is a WebElement in Selenium?
Answer: WebElement
represents an HTML element on a web page. It provides methods to interact with the element, such as click()
, sendKeys()
, and getText()
.
33. How can you verify text on a web page using Selenium?
Answer: You can verify text by retrieving the text from a WebElement
and comparing it with the expected value.
String actualText = driver.findElement(By.id("elementId")).getText();
assertEquals(actualText, "Expected Text");
34. How do you handle a multi-select dropdown in Selenium?
Answer: A multi-select dropdown can be handled using the Select
class and selecting multiple options.
Select multiSelect = new Select(driver.findElement(By.id("multiSelectId")));
multiSelect.selectByVisibleText("Option 1");
multiSelect.selectByVisibleText("Option 2");
35. What is the purpose of the Actions
class in Selenium?
Answer: The Actions
class is used to perform complex user interactions, such as mouse movements, keyboard actions, and drag-and-drop operations.
36. How can you check if an element is present on a web page?
Answer: You can check if an element is present by using findElements()
and checking if the list is not empty.
List<WebElement> elements = driver.findElements(By.id("elementId"));
boolean isPresent = !elements.isEmpty();
37. How do you capture a screenshot of a web page?
Answer: You can capture a screenshot using the TakesScreenshot
interface and save it to a file.
File screenshot = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(screenshot, new File("screenshot.png"));
38. What is the DesiredCapabilities
class used for?
Answer: The DesiredCapabilities
class is used to define the desired capabilities of the browser and platform for the WebDriver instance.
39. How can you handle different frames and iframes in Selenium?
Answer: Use driver.switchTo().frame()
to switch to a specific frame or iframe. Use driver.switchTo().defaultContent()
to switch back to the main content.
40. What is a Wait
in Selenium and why is it important?
Answer: Wait
in Selenium is used to pause the execution of a script until a certain condition is met. It is important for handling dynamic web elements and ensuring that tests run reliably.
41. How do you perform a double-click action in Selenium?
Answer: Use the Actions
class to perform a double-click action.
Actions actions = new Actions(driver);
actions.doubleClick(element).perform();
42. How do you switch to a new window or tab in Selenium?
Answer: Switch to a new window or tab using driver.switchTo().window()
with the handle of the new window.
String originalHandle = driver.getWindowHandle();
for (String handle : driver.getWindowHandles()) {
if (!handle.equals(originalHandle)) {
driver.switchTo().window(handle);
}
}
43. What are the differences between assertEquals
and assertTrue
?
Answer: assertEquals
checks if two values are equal, while assertTrue
checks if a condition is true.
44. How can you handle Ajax-based dynamic content?
Answer: Use WebDriverWait
to wait for specific conditions related to Ajax content, such as the presence or visibility of an element.
45. How do you interact with elements that have dynamic IDs?
Answer: Use other attributes or relative locators to find elements with dynamic IDs, or use XPath/CSS selectors to handle such cases.
46. What is the role of PageFactory
in Selenium?
Answer: PageFactory
is used for initializing web elements in the Page Object Model. It helps in creating a more maintainable and readable codebase.
47. How do you use PageFactory
to initialize elements?
Answer: Use PageFactory.initElements()
to initialize elements in a Page Object class.
PageFactory.initElements(driver, this);
48. How do you handle JavaScript execution errors in Selenium?
Answer: Handle JavaScript execution errors by using proper error handling techniques and validating JavaScript execution using JavascriptExecutor
.
49. What are the common challenges in Selenium automation?
Answer: Common challenges include handling dynamic content, dealing with pop-ups, managing different browser versions, and ensuring test stability.
50. How do you validate if an element is enabled or disabled?
Answer: Use the isEnabled()
method to check if an element is enabled or disabled.
boolean isEnabled = driver.findElement(By.id("elementId")).isEnabled();
51. How do you wait for an element to be clickable?
Answer: Use WebDriverWait
with ExpectedConditions.elementToBeClickable()
to wait until an element is clickable.
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("elementId")));
52. What is the use of the By
class in Selenium?
Answer: The By
class is used to locate web elements using various strategies, such as ID, name, class name, XPath, CSS selectors, and more.
53. How do you handle checkbox and radio button selection?
Answer: Use click()
to select or deselect checkboxes and radio buttons.
driver.findElement(By.id("checkboxId")).click();
driver.findElement(By.id("radioButtonId")).click();
54. How do you handle unexpected alerts or pop-ups in Selenium?
Answer: Handle unexpected alerts using the Alert
interface and try-catch
blocks to manage exceptions.
55. How do you verify the presence of an element using Selenium?
Answer: Verify the presence of an element using findElement()
and checking for its existence.
WebElement element = driver.findElement(By.id("elementId"));
boolean isPresent = (element != null);
56. How do you retrieve an attribute value of an element?
Answer: Use the getAttribute()
method to retrieve the value of an attribute.
String attributeValue = driver.findElement(By.id("elementId")).getAttribute("attributeName");
57. What is the use of the sendKeys()
method?
Answer: The sendKeys()
method is used to input text into text fields, such as login forms, search boxes, and more.
58. How do you handle elements that are hidden or invisible?
Answer: Use WebDriverWait
to wait for the visibility of elements or handle them using appropriate strategies.
59. How can you find elements using CSS Selectors?
Answer: Use the By.cssSelector()
method to locate elements based on CSS attributes.
driver.findElement(By.cssSelector(".className"));
60. How do you execute a test script in multiple browsers?
Answer: Use different WebDriver instances for different browsers, such as ChromeDriver, FirefoxDriver, and EdgeDriver.
61. How do you handle JavaScript alerts, confirms, and prompts?
Answer: Handle JavaScript alerts, confirms, and prompts using the Alert
interface and its methods.
Alert alert = driver.switchTo().alert();
alert.accept(); // For alerts
alert.dismiss(); // For confirms
alert.sendKeys("text"); // For prompts
62. How do you switch to a parent window from a child window?
Answer: Switch to the parent window using driver.switchTo().window()
with the handle of the parent window.
driver.switchTo().window(parentHandle);
63. What is the use of implicit wait
in Selenium?
Answer: Implicit wait
sets a default waiting time for the WebDriver to poll the DOM until the element is found.
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
64. How do you handle dropdown menus that are not standard HTML elements?
Answer: Use JavaScript or the Actions
class to interact with non-standard dropdown menus.
65. What are the different ways to locate elements in Selenium?
Answer: Elements can be located using:
- ID
- Name
- Class Name
- Tag Name
- Link Text
- Partial Link Text
- XPath
- CSS Selector
66. How do you check if an element is displayed on the page?
Answer: Use the isDisplayed()
method to check if an element is visible.
boolean isDisplayed = driver.findElement(By.id("elementId")).isDisplayed();
67. How do you perform a right-click (context click) action?
Answer: Use the Actions
class to perform a right-click action.
Actions actions = new Actions(driver);
actions.contextClick(element).perform();
68. How do you handle multiple windows and tabs in Selenium?
Answer: Manage multiple windows and tabs by switching between them using window handles.
69. What is the purpose of the takeScreenshot()
method in Selenium?
Answer: The takeScreenshot()
method is used to capture the current state of the browser window as an image file.
70. How do you manage cookies in Selenium?
Answer: Use driver.manage().getCookies()
to retrieve cookies and driver.manage().addCookie()
to add cookies.
71. How do you handle AJAX calls in Selenium?
Answer: Use WebDriverWait
to wait for the completion of AJAX calls or changes in the DOM.
72. What is the role of ExpectedConditions
in Selenium?
Answer: ExpectedConditions
provides various conditions to wait for, such as visibility of elements, clickability, presence, and more.
73. How do you handle dynamic web elements with changing IDs?
Answer: Use XPath or CSS selectors with attributes that do not change, or use relative locators.
74. How do you perform a multi-step form submission?
Answer: Automate the process by interacting with each step of the form, filling in details, and submitting each step.
75. What are some common exceptions in Selenium?
Answer: Common exceptions include NoSuchElementException
, TimeoutException
, StaleElementReferenceException
, and ElementNotInteractableException
.
76. How do you handle web table data in Selenium?
Answer: Use XPath or CSS selectors to navigate and extract data from web tables.
77. How do you test responsiveness of a web page in Selenium?
Answer: Test responsiveness by changing the browser window size and verifying the layout and elements.
78. How do you implement data-driven testing in Selenium?
Answer: Implement data-driven testing by reading test data from external sources like Excel, CSV, or databases, and using it in test scripts.
79. What are the different types of locators in Selenium?
Answer: The different types of locators include:
- ID
- Name
- Class Name
- Tag Name
- Link Text
- Partial Link Text
- XPath
- CSS Selector
80. How do you perform a scroll operation in Selenium?
Answer: Perform scroll operations using JavaScript through the JavascriptExecutor
.
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("window.scrollBy(0,500);");
81. What is the WebDriver
interface in Selenium?
Answer: The WebDriver
interface defines the methods used to interact with a web browser, such as get()
, findElement()
, click()
, and sendKeys()
.
82. How do you handle pop-ups generated by JavaScript?
Answer: Handle JavaScript pop-ups using the Alert
interface to accept, dismiss, or interact with them.
83. How can you simulate keyboard actions in Selenium?
Answer: Use the Actions
class to simulate keyboard actions like pressing keys, combinations, or sending special keys.
84. How do you perform a file upload in Selenium?
Answer: Use the sendKeys()
method on the file input element to upload a file.
85. How do you handle hidden elements in Selenium?
Answer: Interact with hidden elements if necessary by using JavaScript or handling visibility dynamically.
86. How do you use the findElements()
method?
Answer: Use findElements()
to return a list of WebElement
objects that match the locator.
List<WebElement> elements = driver.findElements(By.className("className"));
87. How do you verify the title of a web page?
Answer: Use getTitle()
to retrieve and verify the title of the current web page.
String title = driver.getTitle();
assertEquals(title, "Expected Title");
88. How do you handle elements with dynamic attributes?
Answer: Use XPath or CSS selectors with relative locators or other attributes that remain constant.
89. How do you interact with a modal dialog in Selenium?
Answer: Switch to the modal dialog using driver.switchTo().activeElement()
and interact with it.
90. How do you perform cross-browser testing using Selenium?
Answer: Use different browser drivers (e.g., ChromeDriver, FirefoxDriver) and configure tests to run on various browsers.
91. How do you ensure that a test script is robust and maintainable?
Answer: Ensure robustness by using proper wait strategies, handling exceptions, and following design patterns like Page Object Model.
92. How do you use Selenium with a test framework like TestNG?
Answer: Integrate Selenium with TestNG by annotating test methods with @Test
and using TestNG’s features for test execution and reporting.
93. How do you execute a test script on a remote machine?
Answer: Use Selenium Grid or a cloud-based service like Sauce Labs or BrowserStack to run tests on remote machines.
94. How do you handle session management in Selenium?
Answer: Manage sessions by using cookies and session IDs to maintain state across tests.
95. What is the DesiredCapabilities
class used for?
Answer: The DesiredCapabilities
class is used to set various capabilities for the browser, such as browser version, platform, and more.
96. How do you perform a dynamic wait in Selenium?
Answer: Use WebDriverWait
to create dynamic waits that wait for specific conditions before proceeding.
97. How do you check if an element is selected or not?
Answer: Use the isSelected()
method to check if a checkbox or radio button is selected.
boolean isSelected = driver.findElement(By.id("elementId")).isSelected();
98. How do you handle JavaScript-based events in Selenium?
Answer: Use JavascriptExecutor
to trigger JavaScript-based events or interact with elements that rely on JavaScript.
99. How do you create a test report in Selenium?
Answer: Create test reports using test frameworks like TestNG or JUnit, which provide built-in reporting capabilities.
100. How do you troubleshoot common issues in Selenium tests?
Answer: Troubleshoot issues by checking logs, verifying locators, using debugging tools, and ensuring that wait conditions are properly set.
Subscribe to QABash Weekly 💥
Dominate – Stay Ahead of 99% Testers!